Configuring a Table Cell Span Provider
In a custom framework, the
<table>
element can have cells that span over multiple columns and
rows. As explained in Configuring Tables,
you need to indicate Oxygen XML Author a method to determine the cell spanning. If you
use the @rowspan
and @colspan
attributes, Oxygen XML Author can determine the cell spanning automatically. In the following
example, the <td>
element uses the @row_span
and
@column_span
attributes that are not recognized by default. You will need
to implement a Java extension class for defining the cell spanning.
-
Create the class simple.documentation.framework.TableCellSpanProvider. This
class must implement the ro.sync.ecss.extensions.api.AuthorTableCellSpanProvider
interface.
import ro.sync.ecss.extensions.api.AuthorTableCellSpanProvider; import ro.sync.ecss.extensions.api.node.AttrValue; import ro.sync.ecss.extensions.api.node.AuthorElement; public class TableCellSpanProvider implements AuthorTableCellSpanProvider {
-
The init method takes ro.sync.ecss.extensions.api.node.AuthorElement that represents the XML
<table>
element as its argument. In this example, the cell span is specified for each of the cells so you leave this method empty. However, there are cases (such as the CALS table model) when the cell spanning is specified in the<table>
element. In such cases, you must collect the span information by analyzing the<table>
element.public void init(AuthorElement table) { }
-
The getColSpan method is taking as argument the table cell. The table layout
engine will ask this AuthorTableSpanSupport implementation what is the column span
and the row span for each XML element from the table that was marked as cell in the CSS
using the property
display:table-cell
. The implementation is simple and just parses the value of column_span attribute. The method must returnnull
for all the cells that do not change the span specification.public Integer getColSpan(AuthorElement cell) { Integer colSpan = null; AttrValue attrValue = cell.getAttribute("column_span"); if(attrValue != null) { // The attribute was found. String cs = attrValue.getValue(); if(cs != null) { try { colSpan = new Integer(cs); } catch (NumberFormatException ex) { // The attribute value was not a number. } } } return colSpan; }
-
The row span is determined in a similar manner:
public Integer getRowSpan(AuthorElement cell) { Integer rowSpan = null; AttrValue attrValue = cell.getAttribute("row_span"); if(attrValue != null) { // The attribute was found. String rs = attrValue.getValue(); if(rs != null) { try { rowSpan = new Integer(rs); } catch (NumberFormatException ex) { // The attribute value was not a number. } } } return rowSpan; }
-
The method hasColumnSpecifications always returns
true
considering column specifications always available.public boolean hasColumnSpecifications(AuthorElement tableElement) { return true; }
Note: The complete source code for framework customization examples can be found in the oxygen-sample-framework module of the Oxygen SDK, available as a Maven archetype on the Oxygen XML Author website. -
In the listing below, the XML document contains the table element:
<table> <header> <td>C1</td> <td>C2</td> <td>C3</td> <td>C4</td> </header> <tr> <td>cs=1, rs=1</td> <td column_span="2" row_span="2">cs=2, rs=2</td> <td row_span="3">cs=1, rs=3</td> </tr> <tr> <td>cs=1, rs=1</td> </tr> <tr> <td column_span="3">cs=3, rs=1</td> </tr> </table>
When no table cell span provider is specified, the table has the following layout:
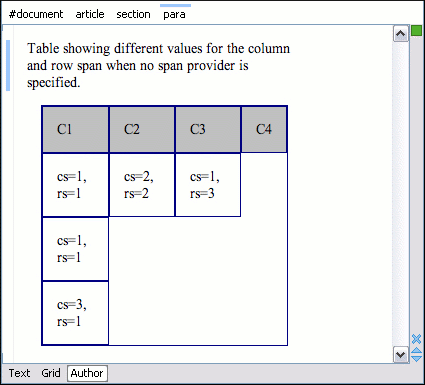
When the above implementation is configured, the table has the correct layout:
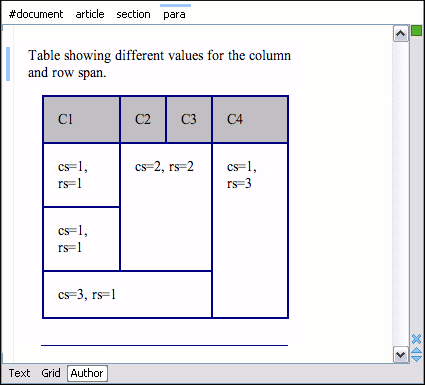